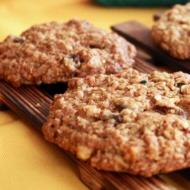
Two-dimensional arrays. Filling a two-dimensional array according to a given rule
Sections: Computer science
Subject: Two-dimensional arrays. Filling a two-dimensional array according to a given rule.
Goals: practice skills of working with elements of a two-dimensional array, learn to fill two-dimensional arrays according to a given rule, learn to derive the relationship between the row number and column number; development of students' logical thinking.
PROGRESS OF THE CLASS
1. Updating knowledge
Arrays in which the position of elements is described by two indices are called two-dimensional. The structure of such an array can be represented by a rectangular matrix. Each element of the matrix is uniquely identified by indicating the row and column number, the row number is i, the column number is j.
Consider matrix A of size n*m:
a 11 | a 12 | a 13 | a 14 |
a 21 | a 22 | a 23 | a 24 |
a 31 | a 32 | a 33 | a 34 |
Matrix of 3 rows and 4 columns, number of rows n=3, number of columns m=4. Each element has its own number, which consists of two numbers - the number of the row in which the element is located, and the number of the column. For example, a23 is an element found in the second row and third column.
A two-dimensional array in Turbo Pascal can be described in different ways. To describe a two-dimensional array, you need to determine what type of its elements are and how they are numbered (what type of index it is). There are several ways to describe a two-dimensional array.
Const maxN=…; (Maximum row counts)
maxM=…; (Maximum number of columns)
1 way
Type Mas = array of<тип элементов>; (One dimensional array)
Type TMas = array of Mas; (One-dimensional array whose elements are one-dimensional arrays)
Method 2
Type TMas = array of array of<тип
элементов>;
(One-dimensional array whose elements are one-dimensional arrays)
3 way
Type<имя типа>= array of<тип элементов>; (Two-dimensional array)
Preference is given to the third method of describing a two-dimensional array.
For example:
Const N=3; M=4;
Type TMas= array of integer; (2-dimensional array of integers)
The formation of a two-dimensional array can be carried out in four ways: input from the keyboard, through a random number generator, according to a given rule, or using a file.
1) Formation of a two-dimensional array using keyboard input and an algorithm for row-by-row output of matrix elements.
Const N=10;M=10;
Type Tmas= array of integer;
Var A:Tmas; i,j:integer;
Begin
(Input of matrix elements)
For i:=1 to N do
For j:=1 to M do
Read(A);
(Output of matrix elements)
For i:=1 to N do begin
For j:=1 to M do
Write(A:4); (First line is printed)
Writeln (New line)
end;
End.
2) A fragment of a program for generating a two-dimensional array through a random number generator.
Begin
Randomize; (Initializing the random number generator)
(Input of matrix elements)
For i:=1 to N do
For j:=1 to M do
A:=random(45)-22;
2. Studying new material. Filling an array according to a rule
Let's consider several fragments of programs for filling a two-dimensional array according to a certain law. To do this, you need to derive a filling rule.
1. Fill array A of size n*m as follows, for example
1
2
3
4
5
6
7 8
16 15
14 13
12 11
10 9
17 18
19 20
21 22
23 24
32 31
30 29
28 27
26 25
33 34
35 36
37 38
39 40
48 47
46 45
44 43
42 41
The array is filled according to the “snake” principle. Filling rule: if the line number is an odd number, then A=(i-1)*m+j, otherwise A=i*m-j+1.
program M1A;
n,m,i,j: integer;
begin
readln(n,m);
for i:=1 to n do begin
for j:=1 to m do
begin
if i mod 2 = 1 then
A=(i-1)*m+j
else
A=i*m-j+1;
write(A:3);
end;
writeln;
end;
readln;
end.
Here is an example of a program for another method of filling according to a given rule:
program M1B;
var A:array of integer;
n,m,i,j: integer;
c:integer;
begin
readln(n,m);
c:=1;
for i:=1 to n do
begin
for j:=1 to m do
begin
A:=c;
if (i mod 2 = 0) and (j<>m)then
dec(c)
else
inc(c);
write(A:3);
end;
c:=c+m-1;
writeln;
end;
readln;
end.
2. Fill array A according to the following principle:
1
0
2
0
3
0 4
0
5
0
6
0
7 0
8
0
9
0
10
0 11
0
12
0
13
0
14 0
program M2;
var A:array of integer;
n,m,i,j: integer;
c:integer;
begin
readln(n,m);
c:=0;
for i:=1 to n do
begin
for j:=1 to m do
begin
if (i-1+j) mod 2 = 0 then
A:=0
else
begin
inc(c);
A:=c;
end;
write(A:5);
end;
writeln;
end;
readln;
end.
3. Fill array A according to the following principle:
1
12 13
24 25
36
2
11 14
23 26
35
3
10 15
22 27
34
4
9
16 21
28 33
5
8
17 20
29 32
6
7
18 19
30 31
var A:array of integer;
n,m,i,j: integer;
c:integer;
begin
readln(n,m);
c:=1;
for j:=1 to m do
begin
for i:=1 to n do
begin
A:=c;
if (j mod 2 = 0) and (i<>n)then
dec(c)
else
inc(c);
end;
c:=c+n-1;
end;
for i:=1 to n do
begin
for j:=1 to m do
write(A:5);
writeln;
end;
readln;
end.
4. Fill array A according to the following principle:
1
2
3
4 5
2
3
4
5 1
3
4
5
1 2
4
5
1
2 3
5
1
2
3 4
var i,j,m,c,d: integer;
begin
c:=1;
readln(m);
for j:=1 to m do
begin
i:=c;
d:=1;
repeat
A:=d;
inc(i);
if i>m then
i:=1;
inc(d);
until i=c;
dec(c);
if c<= 0 then
c:=m-c;
end;
for i:=1 to m do
begin
for j:=1 to m do
write(A:2);
writeln;
end;
end.
5. Fill array A according to the following principle:
1
0
0
0 1
0
1
0
1 0
0
0
1
0 0
0
1
0
1 0
1
0
0
0 1
var m,i,j: integer;
A:array of integer;
begin
readln(m);
for i:=1 to m do
begin
for j:=1 to m do
begin
if (i=j) or (m-i+1=j) then
A:=1
else
A:=0;
write(A:2);
end;
writeln;
end;
end.
3. Problems for independent solution
6
5
4
3
2 1
7
8
9
10 11
12
18 17
16 15
14 13
19 20
21 22
23 24
30 29
28 27
26 25
31 32
33 34
35 36
36
25 24
13 12
1
35 26
23 14
11 2
34 27
22 15
10 3
33 28
21 16
9 4
32 29
20 17
8 5
31 30
19 18
7 6
0
1
1
1 0
1
0
1
0 1
1
1
0
1 1
1
0
1
0 1
0
1
1
1 0
4) Fill the array according to the following principle:
31
32 33
34 35
36
25 26
27 28
29 30
19 20
21 22
23 24
13 14
15 16
17 18
7
8
9
10 11
12
1
2
3
4
5 6
5) Fill the array according to the following principle:
31
25 19
13
7 1
32 26
20 14
8 2
33 27
21 15
9 3
34 28
22 16
10 4
35 29
23 17
11 5
36 30
24 18
12 6
Homework:
1) Fill the array according to the following principle:
6
7
18 19
30 31
5
8
17 20
29 32
4
9
16 21
28 33
3
10 15
22 27
34
2
11 14
23 26
35
1
12 13
24 25
36
2) Fill the array according to the following principle:
31
32 33
34 35
36
30 29
28 27
26 25
19 20
21 22
23 24
18 17
16 15
14 13
7
8
9
10 11
12
6
5
4
3
2 1
3) Fill the array according to the following principle:
0
1
1
1 0
1
0
1
0 1
1
1
0
1 1
1
0
1
0 1
0
1
1
1 0
Sections: Computer science
Goals:
- Introduce students to the possibility of filling and processing an array.
- Create a graphical interface for a project to fill an array and calculate the sum of elements in a given array.
- Develop cognitive interest in the subject
- Foster a responsible attitude towards learning
DURING THE CLASSES
1. Updating the lesson
Organizing time
Frontal survey on the previous topic “The concept of an array. One-dimensional array"
2. Formation of skills and abilities
Explanation of new material
Array Declaration
Declaring an array is similar to declaring variables; you only need to specify the range of index changes. For example, declaring a one-dimensional integer array containing 10 elements is done as follows:
A: array of integer;
Basic tasks when working with arrays
1. Formation (filling) of the array
1.1. according to the formulas For i:=1 to 10 do a[i]:= i*i;
1.2. generate randomly For i:=1 to 10 do a[i]:= random(20):
The built-in function RANDOM(MAX), returns a random integer uniformly distributed in the range from 0 to MAX – 1 (MAX is the access parameter)
1.3. enter from the keyboard For i:=1 to 10 do read(a[i]);
2. Sort the array (ascending, descending);
3. Search for elements in an array;
4. Selecting elements from an array by condition;
Filling the array randomly.
To start working with an array, you need to fill it, i.e. assign specific values to array elements. To generate a sequence of random numbers, we use the Random(100) function. When you run the program, this function will output a pseudo-random sequence of integers in the range from 0 to 100.
To generate sequences of random numbers that differ from each other, it is recommended to use the Randomize operator
Actions with one-dimensional arrays
1. Calculation of the sum of elements
For I:= 1 To 10 Do s:=s+ a[i]; (usual accumulation of amount in s)
2. Calculation of the product
For I:= 1 To 10 Do р:=р* a[i]; (usual accumulation of product in p)
3. Search for an element with a given value
3. Note Development of skills and abilities in practice
Project “Sum of elements in an array”. Let's develop a project “Sum of elements in an array”, which will fill the array with random numbers and calculate the sum of these numbers
First, let's create a procedure for filling the array
1.Launch the Delphi programming system.
2. Work on the project begins with creating a graphical interface, for this in the window Form Builder Control elements are placed on the form. To create a graphical interface for the project, we will place on the form two text fields for displaying numerical data (one for filling an array, the other for displaying a sum) and two buttons for implementing event procedures: filling an array and sum
3. With Toolbars place an Editl text field and a Buttonl command button on the Forml
The next step is to create event procedure code. Double-clicking the button for which you need to create program code brings up a window Program code with an empty event procedure template.
4. Double-click on the Buttonl button, a template event procedure TForml.ButtonlClick will appear: Declare an array A and description of variables I, S in the var variable description section
A:array of integer;
procedure TForm1.Button1Click(Sender: TObject);
For I:= 1 To 10 Do
A[I] := Random(10);
Edit1.Text:= Edit1.Text +" " + IntToStr(a[i]);
5. Save Project As
6. Compiling the project (Project - Compile)
Now let's create a procedure to calculate the sum of the elements in the filled array
By using Toolbars Let's place a button Button2 and a text field Edit2 on the Forml. Double-clicking the button Button2, for which you need to create a program code, opens a window Program code with an empty event procedure template.
procedure TForm1.Button2Click(Sender: TObject);
For I:= 1 To 10 Do
Edit2.Text:= Edit2.Text +" " + IntToStr(s)
Saving the project of the entire project (Save Project).
Let's compile the project (by pressing the F9 key).
Click the Fill Array and Sum buttons.
The results of the amounts for various filling options will be displayed in the text field
4. Summing up
5. Homework: Create a project “Product of array elements”, which involves filling an array with random numbers and the ability to display the product of all elements in the array in a text field.
One-dimensional arrays. Forming an array and displaying its elements
Definition of the concept
An array is a collection of data of the same type with a common name for all elements.
The elements of the array are numbered, and each of them can be accessed by number. The numbers of array elements are otherwise called indices, and the array elements themselves are called indexed variables.
a[n] |
||||||
- 0. 5 |
-5.2 |
… |
0.6 |
Vector (linear or one-dimensional array) is an example of an array in which the elements are numbered with a single index.
- As numbers (index) array element, in general, the expression is used ordinal type(most often this is an integer constant or a variable of integer type: integer, word, byte or shortint)
- When accessing an array element, the index is indicated in square brackets. For example, a, mass.
- Array elements are processed when element indices change. For example, when using an expression, the following variables are useful for looping through the elements of an array:
- a[i] - all elements;
- a - elements located in even places;
- a - elements in odd places
Array description
- Defining a variable as an array without first declaring the array type
vara,b,c: array of integer; var s: array of integer; k: array of integer; |
Note
- The array description is required by the compiler to allocate memory for its elements.
- A variable is defined as an array using a function word array(array). The range is indicated in square brackets, i.e. the lower and upper bounds of the array index value. The upper limit value cannot be less than the lower limit.
- Here the variables s and k are considered to be of different types. To ensure compatibility, it is necessary to use variable declarations through a preliminary type declaration.
- If the types of arrays are identical, then in a program one array can be assigned to another. In this case, the values of all variables in one array will be assigned to the corresponding elements of the second array.
- There are no relational operations defined on arrays. You can only compare two arrays element by element.
- Preliminary description of the array type
const n = 5; type mas = array of integer; var a: mas; |
Note
- The elements of the array will be accessed like this: a, a, a, a, a (i.e. the array contains five elements).
- Using constants(in this example, n) when describing an array is preferable, since if the size of the array changes, there will be no need to make corrections throughout the program text.
- Setting an Array to a Typed Constant
const x: array of integer = (1, 2, 3, 4, 5, 6); |
Note
- In this example, not only is memory allocated for the array, but the cells are also filled with data.
- Array elements can be change during the program (like all typed constants).
Filling an Array with Data
§ To fill an array data (and its output), a loop with a parameter is most often used for
§ To fill an array with random numbers, use the function random and procedure randomize(initializing the random number generator). The recording format is: random(B - A) + A, where A and B are taken from the interval :4);
Example program for input and output of an array
Formulation of the problem. Get the sum of the elements of an array consisting of 10 integer elements. Array elements are entered from the keyboard.
program array2; var sum: integer; i: byte; a: array of word; begin sum:= 0; fori:= 0 to 9 do begin write("a[",i," ] = "); readln(a[i]); sum:= sum + a[i] end; writeln("sum =", sum) end. |
An example program for working with array elements
Formulation of the problem. Get the arithmetic mean of the array elements. The array elements are filled with random numbers.
program array3; const n = 100; var sar: real; sum: integer; i: byte; a: array of integer; begin sum:= 0; randomize; fori:= 0 to n do begin a[i] := random(100); sum:= sum + a[i] end; sar:= sum/n; writeln("sar =", sar) end. |
The lesson explains how to work with one-dimensional arrays in Pascal, how to use a random number generator - the function random in Pascal. An example of how to derive Fibonacci numbers is considered.
The materials on the site are aimed at practical mastery of the Pascal programming language. Brief theoretical information does not claim to be a complete coverage of the material on the topic; The necessary information can be found in large quantities on the Internet. Our tasks include providing the opportunity to gain practical programming skills in Pascal. Solved visual examples and tasks are presented in order of increasing complexity, which will make it easy to study the material from scratch.
Array Declaration
There are two types of arrays in Pascal: one-dimensional and two-dimensional.
Defining a one-dimensional array in Pascal sounds like this: a one-dimensional array is a certain number of elements belonging to the same data type, which have the same name, and each element has its own index - a serial number.
The description of an array in Pascal (declaration) and access to its elements is as follows:
Array Declaration
var length: array [ 1 .. 3 ] of integer ; begin length[ 1 ] : = 500 ; dlina[ 2 ] : = 400 ; dlina[ 3 ] : = 150 ; ... var length: array of integer; begin length:=500; length:=400; length:=150; ...
Announce the size can be used through a constant:
Initializing an Array
In addition, the array itself can be constant, i.e. all its elements in the program are predetermined. The description of such an array looks like this::
const a: array [ 1 .. 4 ] of integer = (1 , 3 , 2 , 5 ) ; |
const a:array of integer = (1, 3, 2, 5);
Filling with consecutive numbers:
Result: A = 8, A = 9, A = 10, ..., A[N] = A + 1
Keyboard input:
Example: Let's look at how an array is entered in Pascal:
writeln("enter the number of elements: "); readln(n); (if the quantity is not known in advance, we ask for it) for i:= 1 to n do begin write("a[", i, "]="); read(a[i]); ...end; ...
✍ Example result:
Enter the number of elements: 3 a=5 a=7 a=4
Printing Array Elements
Example: Let's look at how to display an array in Pascal:
1 2 3 4 5 6 7 8 9 10 11 12 13 | var a: array [ 1 .. 5 ] of integer ; (array of five elements) i:integer ; begin a[ 1 ] : = 2 ; a[ 2 ] : = 4 ; a[ 3 ] : = 8 ; a[ 4 ] : = 6 ; a[ 5 ] : = 3 ; writeln("Array A:"); for i : = 1 to 5 do write (a[ i] : 2 ) ; (output of array elements) end. |
var a: array of integer; (array of five elements) i: integer; begin a:=2; a:=4; a:=8; a:=6; a:=3; writeln("Array A:"); for i:= 1 to 5 do write(a[i]:2); (output array elements) end.
✍ Example result:
Array A: 2 4 8 6 3
To work with arrays, it is most often used in Pascal with a parameter, since you usually know how many elements are in the array, and you can use a loop counter as indices of the elements.
Array 0 task. You must specify a real array of dimensions 6 (i.e., six elements); fill the array with input values and display the elements on the screen. Use two loops: the first for inputting elements, the second for output.
In this example of working with a one-dimensional array, there is an obvious inconvenience: assigning values to elements.
Processing arrays in Pascal, as well as filling an array, usually occurs using a for loop.
Random function in Pascal
In order not to constantly request the values of array elements, a random number generator in Pascal is used, which is implemented by the Random function. In fact, pseudo-random numbers are generated, but that’s not the point.
To generate numbers from 0 to n (not including the value of n itself, integers in the interval of integer; i:integer; begin randomize; for i:=1 to 10 do begin f[i]:=random(10); ( interval ) write(f[i]," "); end; end.
✍ Example result:
9 8 9 2 0 3 6 9 5 0
For real numbers in the interval and display elements on the screen: define three positions for displaying each element.
Fibonacci numbers in Pascal
The most common example of working with an array is the output of a series of Fibonacci numbers in Pascal. Let's consider it.
Example: Fibonacci number series: 1 1 2 3 5 8 13…
f[ 0 ] : = 1 ; f[ 1 ] : = 1 ; f[ 2 ] : = 2 ; ... f:=1; f:=1; f:=2; ...
f[ 2 ] : = f[ 0 ] + f[ 1 ] ; f[ 3 ] : = f[ 1 ] + f[ 2 ] ;
f[ i] : = f[ i- 2 ] + f[ i- 1 ] ; f[i]:=f+f;
We received the formula for the elements of the series.
Example: Calculate and print the first 20 Fibonacci numbers.
1 2 3 4 5 6 7 8 9 10 11 | var i: integer ; f: array [0 .. 19] of integer; begin f[ 0 ] : = 1 ; f[ 1 ] : = 1 ; for i: = 2 to 19 do begin f[ i] : = f[ i- 1 ] + f[ i- 2 ] ; writeln (f[ i] ) end ; end. |
var i:integer; f:arrayof integer; begin f:=1; f:=1; for i:=2 to 19 do begin f[i]:=f+f; writeln(f[i]) end; end.
In this example, the principle of working with number series becomes clear. Usually, to derive a number series, a formula is found for determining each element of this series. So, in the case of Fibonacci numbers, this formula-rule looks like f[i]:=f+f. Therefore, it must be used in a for loop when forming array elements.
Array 2 task. Given a row of 10 arbitrary numbers: a, a, ... , a (use the random() function). Calculate and print the sum of triplets of adjacent numbers: a+a+a , a+a+a , a+a+a , …… , a+a+a
Pseudocode:
Finding the maximum element by its index:
Array_min task: Find the minimum element of the array. Print the element and its index.
Array 4 task. Given an array of 10 integer elements. Find the number of negative ones and display the number on the screen.
Array 5 task. Find the minimum and maximum of n entered numbers (array). Determine the distance between these elements. 3 2 6 1 3 4 7 2 >>> min=1, max=7, distance=3
Array 6 task. Given an integer array of size N. Print all even numbers contained in this array in descending order of their indices, as well as their number K. N=4 mas: 8 9 2 5 >>> 2 8 quantity= 2
Array 7 task. Enter an array of 5 elements from the keyboard, find the two maximum elements and their numbers in it.
Example:
Initial array: 4 -5 10 -10 5 maximum A=10, A=5
Search in an array
Let's look at a complex example of working with one-dimensional arrays:
Example: Given an array of 10 numbers. Determine whether the number entered by the user is in the array. If there is, output "found", if not - "not found".
The difficulty of the task is that to print the words "found" or "not found" needed once.
To solve this problem, you will need a break statement to exit the loop.
Solution Option 1. For loop:
Show solution
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | var f: array [ 1 .. 10 ] of integer ; flag:boolean ; i, c: integer ; begin randomize; for i: = 1 to 10 do begin f[ i] : = random(10 ) ; write (f[ i] , " " ) ; end ; flag: = false ; writeln( "enter sample") ; readln(c); for i: = 1 to 10 do if f[ i] = c then begin writeln ("found" ) ; flag: = true ; break ; end ; if flag= false then writeln ("not found" ) ; end. |
var f: array of integer; flag:boolean; i,c:integer; begin randomize; for i:=1 to 10 do begin f[i]:=random(10); write(f[i]," "); end; flag:=false; writeln("enter sample"); readln(c); for i:=1 to 10 do if f[i]=c then begin writeln("found"); flag:=true; break; end; if flag=false then writeln("not found"); end.
Let's consider effective solution:
Task: find an element equal to X in the array, or establish that it does not exist.
Algorithm:
- start from 1st element (i:=1);
- if the next element (A[i]) is equal to X , then finish the search otherwise go to the next element.
solution in Pascal Option 2. While loop:
We invite you to watch a detailed video analysis of searching for an element in an array (an effective algorithm):
Array 8 task. Fill an array of 10 elements with random numbers in the interval and print the numbers of all elements equal to X .
Example:
Initial array: 4 0 1 2 0 1 3 4 1 0 What are we looking for? 0 A, A, A
Cyclic shift
Example: shift the array elements to the left by 1 position, the first element replaces the last one.
Solution:
Algorithm:
A:=A; A:=A;... A:=A[N];
Program:
Array 9 task. Fill an array of 10 elements with random numbers in the interval [-10..10] and perform a cyclic shift to the left without first element.
Example: Initial array: 4 -5 3 10 -4 -6 8 -10 1 0 Result: 4 3 10 -4 -6 8 -10 1 0 -5
Rearranging elements in an array
Let's look at how an array is rearranged or reversed.
Example: rearrange array elements in reverse order
Solution:
Algorithm:
Pseudocode:
Program:
Array 10 task. Fill an array of 10 elements with random numbers in the interval [-10..10] and reverse all elements except the last one.
Example: Source array: -5 3 10 -4 -6 8 -10 1 0 4 Result: 0 1 -10 8 -6 -4 10 3 -5 4
Selecting elements and saving to another array
Example: find elements in an array that satisfy some condition (for example, negative) and copy them to another array
Solution:
Solution: count the number of elements found using the count counter, install the next element in place B. Using a variable count must be assigned 1 .
Output of array B:
writeln("Selected items"); for i:=1 to count-1 do write(B[i], " ")
Array task 11. Fill an array with random numbers in the interval and write into another array all the numbers that end in 0.
Example: Source array: 40 57 30 71 84 Ends with 0: 40 30
Sorting Array Elements
Bubble sorting
- In this type of sorting, the array is represented as water, small elements are bubbles in the water that float to the top (the lightest).
- During the first iteration of the loop, the elements of the array are compared with each other in pairs: the penultimate with the last, the penultimate with the penultimate, etc. If the previous element turns out to be larger than the subsequent one, then they are exchanged.
- During the second iteration of the loop, there is no need to compare the last element with the penultimate one. The last element is already in place, it is the largest. This means that the number of comparisons will be one less. The same goes for each subsequent iteration.
Execution in Pascal:
1 2 3 4 5 6 7 8 | for i: = 1 to N- 1 do begin for j: = N- 1 downto i do if A[ j] > A[ j+ 1 ] then begin with : = A[ j] ; A[ j] : = A[ j+ 1 ] ; A[ j+ 1 ] : = с; end ; end ; |
for i:=1 to N-1 do begin for j:=N-1 downto i do if A[j] > A then begin from:= A[j]; A[j] := A; A := c; end; end;
Array 12 task. Fill an array of 10 elements with random numbers in the interval and sort the first half of the array in ascending order, and the second in descending order (using the ‘Bubble’ method). Example: Source array: 14 25 13 30 76 58 32 11 41 97 Result: 13 14 25 30 76 97 58 41 32 11
Sorting by selection
- the minimum element is searched in the array and placed in first place (switched places with A);
- Among the remaining elements, a search is also made for the minimum one, which is placed in second place (switched places with A), etc.
for i:= 1 to N-1 do begin min:= i ; for j:= i+1 to N do if A[j]< A then min:=j; if min <>i then begin c:=A[i]; A[i]:=A; A:=c; end; end;
Array 13 task: Fill an array of 10 elements with random numbers in the interval and sort it in ascending order of the sum of the digits Example: Source array: 14 25 13 12 76 58 21 87 10 98 Result: 10 21 12 13 14 25 76 58 87 98
Quick sort or quick sort
Algorithm:
Execution in Pascal:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | procedure QSort ( first, last: integer ) ; var L, R, c, X: integer ; begin if first< last then begin X: = A[ (first + last) div 2 ] ; L: = first; R: = last; while L <= R do begin while A[ L] < X do L: = L + 1 ; while A[ R] >X do R: = R - 1 ; if L<= R then begin c: = A[ L] ; A[ L] : = A[ R] ; A[ R] : = c; L: = L + 1 ; R: = R - 1 ; end ; end ; QSort(first, R) ; QSort(L, last) ; end ; end . |
procedure QSort(first, last: integer); var L, R, c, X: integer; begin if first< last then begin X:= A[(first + last) div 2]; L:= first; R:= last; while L <= R do begin while A[L] < X do L:= L + 1; while A[R] >X do R:= R - 1; if L<= R then begin c:= A[L]; A[L]:= A[R]; A[R]:= c; L:= L + 1; R:= R - 1; end; end; QSort(first, R); QSort(L, last); end; end.
Array 14 task:
Fill an array of 10 elements with random numbers in the range [-50..50] and sort it using the quick sort algorithm.
1 Method (keyboard filling. Dynamicinputdata)
M:array of integer;
For I:=1 To 10 Do Begin
Write("Enter ",I," value ");
2 Method (using a random number generator)
M: array of integer;
For I:=1 To 25 Do Begin
M[I]:=Random(50);
3 Method (static data entry)
M: array of integer = (31,28,31,30,31,30,31,31,30,31,30,31);
For I:=1 To 9 Do
1.4 Examples of problem solving
1. Algorithms for searching and assigning values to array elements
1. Create a program for processing an array of dimension n filled with integers entered from the keyboard. Print the indices and values of positive array elements.
A:ARRAY OF INTEGER;
(Filling the array)
FOR I:=1 TO N DO Begin
Write("Enter ",I," array element "); ReadLn(A[I]);
(Processing Array Elements)
FOR I:=1 TO N DO
IF A[I]>0 THEN WriteLn("Positive element = ",A[I]," its index = ",I);
2. Create a program for calculating and printing the values of the function Y=sin(x-1)/2x. Set the argument values in an array X consisting of 6 elements. Write the function values into the Y array.
X,Y:ARRAY OF REAL;
FOR I:=1 TO 6 DO Begin
Write("Enter ",I," argument value "); ReadLn(X[I]);
FOR I:=1 TO 6 DO Begin
Y[I]:=SIN(X[I]-1)/(2*X[I]);
WriteLn(" X= ",X[I]:4:1," Y=",Y[I]:5:2);
3. Given an array M, consisting of 30 elements. Array elements are arbitrary integers. Display the value of every fifth and positive element. Output the specified elements into a string.
M:ARRAY OF INTEGER;
ClrScr; Randomize;
WriteLn("Array element values");
FOR I:=1 TO 30 DO Begin
M[I]:=Random(20)-4; Write(M[I]:3);
WriteLn("Values of every fifth and positive array element");
While I<=30 DO Begin
IF M[I] > 0 THEN Write(M[I]:3);
Examples for independent solutions:
Given a one-dimensional array of dimension 10, filled with integers entered from the keyboard, and the value N. Replace negative elements with N. Display the modified array in one line.
Given a one-dimensional array of dimension N, filled with random numbers in the range from -15 to 20. Display the values of array elements whose absolute value is >10.
Given a one-dimensional array of dimension N, filled with random numbers. Square every third element of the array if the element is negative. Display the modified array on the screen.
Create a program for calculating and printing the values of the function Y=(sinx+1)cos4x. Set the argument values in an array X consisting of 10 elements. Write the function values into the Y array.
From the elements of array A, consisting of 25 elements, form array D of the same dimension according to the rule: the first 10 elements are found according to the formula Di=Ai+i, the rest - according to the formula Di=Ai-i.